System
- The System class holds a collection of Static methods and variables.
The standard input, output, and error output of the Java run time are stored in the in, out, and err variables.
The methods defined by the System are as follows where most of them throws SecurityException if the operation is not permitted by the manager.
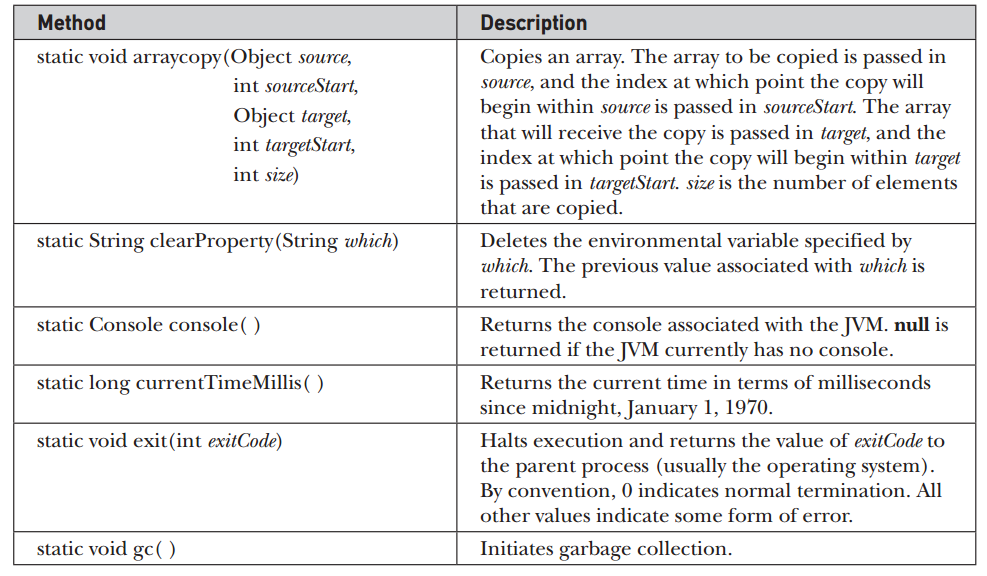
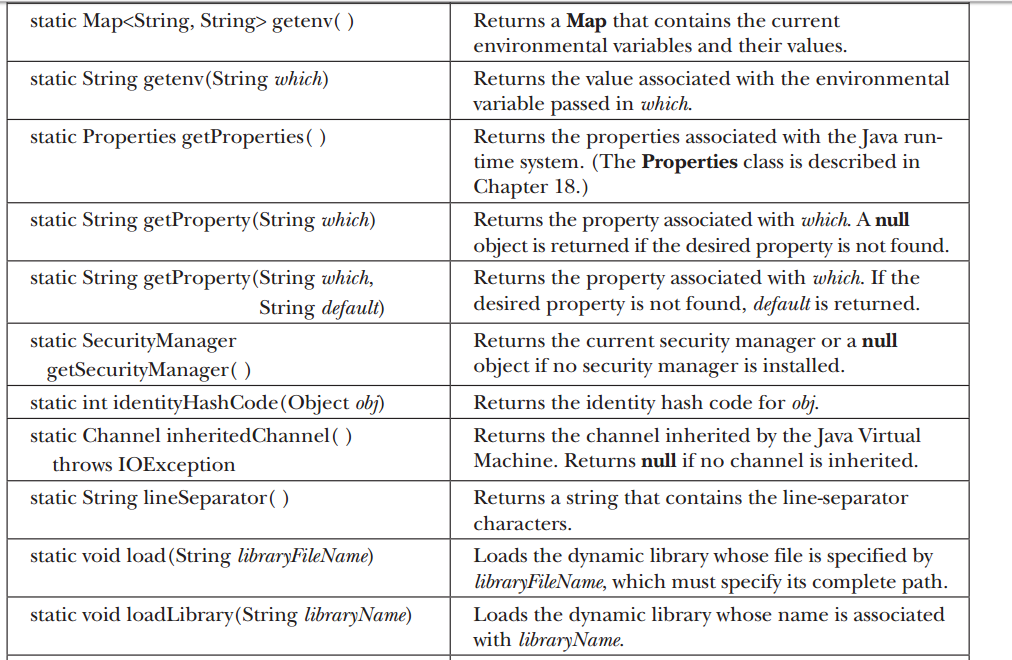
currentTimeMillis( )
- This is the method of System class which returns the time taken for executing a various parts of program in milliseconds.
// Timing program execution.
public class Elapsed
{
public static void main(String args[])
{
long start, end;
System.out.println("Timing a for loop from 0 to 100,000,000");
// time a for loop from 0 to 100,000,000
start = System.currentTimeMillis(); // get starting time
System.out.println("start time is::"+start);
for(long i=0; i < 100000000L; i++) ;
end = System.currentTimeMillis(); // get ending time
System.out.println("End time is::"+end);
System.out.println("Elapsed time: " + (end-start));
}
}
Output:
Timing a for loop from 0 to 100,000,000
start time is::1496659694927
End time is::1496659694988
Elapsed time: 61
- We can use nanoTime() method to get the time in nanoseconds which is depends on the system that allows or not.
// Timing in nanoseconds program execution.
public class Elapsed
{
public static void main(String args[])
{
long start, end;
System.out.println("Timing a for loop from 0 to 100,000,000");
// time a for loop from 0 to 100,000,000
start = System.nanoTime(); // get starting time
System.out.println("start time is::"+start);
for(long i=0; i < 100000000L; i++) ;
end = System.nanoTime(); // get ending time
System.out.println("End time is::"+end);
System.out.println("Elapsed time: " + (end-start));
}
}
Output:
Timing a for loop from 0 to 100,000,000
start time is::21822483561178078
End time is::21822483621049032
Elapsed time: 59870954
// Using arraycopy().
public class ACDemo
{
static byte a[] = { 65, 66, 67, 68, 69, 70, 71, 72, 73, 74 };
static byte b[] = { 77, 77, 77, 77, 77, 77, 77, 77, 77, 77 };
public static void main(String args[])
{
System.out.println("a = " + new String(a));
System.out.println("b = " + new String(b));
System.arraycopy(a, 0, b, 0, a.length);
System.out.println("a = " + new String(a));
System.out.println("b = " + new String(b));
System.arraycopy(a, 0, a, 1, a.length - 1);
System.arraycopy(b, 1, b, 0, b.length - 1);
System.out.println("a = " + new String(a));
System.out.println("b = " + new String(b));
}
}
Output:
a = ABCDEFGHIJ
b = MMMMMMMMMM
a = ABCDEFGHIJ
b = ABCDEFGHIJ
a = AABCDEFGHI
b = BCDEFGHIJJ
Environment Properties
- The following are the properties which are available in all cases.
- We can obtain the value of these properties by calling System.getProperty( ) method.
public class SystemDemo {
public static void main(String[] args) {
// prints the name of the system property
System.out.println(System.getProperty("user.dir"));
// prints the name of the Operating System
System.out.println(System.getProperty("os.name"));
// prints Java Runtime Version
System.out.println(System.getProperty("java.runtime.version" ));
}
}
Output:
/web/com/1496661268_134943
Linux
1.8.0_65-b17